Data Science with Python
Data Science with Python Course Guide
1. Introduction to Data Science
1.1 What is Data Science?
Data Science is a field that uses different methods to get useful information from data. It combines math, computer science, and specific knowledge to solve problems and make decisions.
1.2 Applications of Data Science in Various Domains
Data Science is used in many areas, such as:
- Business: To understand customer behavior and improve sales
- Healthcare: To predict diseases and create better treatments
- Finance: To detect fraud and manage risks
- Transportation: To make traffic flow better and plan routes
1.3 Role of a Data Scientist
A Data Scientist does many things:
- Collects and cleans data
- Looks for patterns in data
- Creates models to predict future trends
- Shares findings with others in a clear way
1.4 Data Science vs. Data Analytics vs. Machine Learning
These terms are related but different:
- Data Science: A broad field that includes collecting, analyzing, and using data
- Data Analytics: Focuses on finding insights from existing data
- Machine Learning: A part of Data Science that helps computers learn from data without being specifically programmed
- Getting Started with Python for Data Science
2.1 Installing Python & Jupyter Notebook (Anaconda)
To start using Python for Data Science:
- Download Anaconda from the official website
- Install Anaconda on your computer
- Open Jupyter Notebook from the Anaconda Navigator
2.2 Python Basics: Variables, Data Types, Operators
Learn the basics of Python:
- Variables: How to store data
- Data Types: Numbers, strings, lists, and more
- Operators: How to do math and compare things in Python
2.3 Control Structures: Loops & Conditional Statements
Understand how to control the flow of your program:
- If statements: Make decisions in your code
- For loops: Repeat actions a certain number of times
- While loops: Repeat actions until a condition is met
2.4 Functions & Modules in Python
Learn how to organize and reuse your code:
- Functions: Write reusable pieces of code
- Modules: Use pre-written code to save time
- Data Handling & Manipulation with Pandas & NumPy
3.1 Introduction to NumPy: Arrays & Operations
NumPy is a library for working with numbers in Python:
- Create arrays
- Do math with arrays
- Reshape and combine arrays
3.2 Pandas DataFrames & Series
Pandas helps you work with structured data:
- Create DataFrames and Series
- Read data from files
- Select and filter data
3.3 Data Cleaning & Preprocessing
Learn how to make your data ready for analysis:
- Remove duplicates
- Fix formatting issues
- Handle outliers
3.4 Handling Missing Values & Duplicates
Discover ways to deal with incomplete data:
- Find missing values
- Decide whether to remove or fill in missing data
- Remove duplicate entries
- Data Visualization with Matplotlib & Seaborn
4.1 Plotting Graphs using Matplotlib
Matplotlib helps you create basic graphs:
- Line plots
- Bar charts
- Scatter plots
4.2 Advanced Visualization using Seaborn
Seaborn makes your graphs look better:
- Heatmaps
- Pairplots
- Box plots
4.3 Interactive Visualizations with Plotly
Plotly lets you create graphs you can interact with:
- Zoom in and out
- Hover over data points for more information
- Create animations
- Exploratory Data Analysis (EDA)
5.1 Understanding Dataset Characteristics
Learn how to get to know your data:
- Look at the first few rows
- Check data types
- Count unique values
5.2 Descriptive Statistics & Summary Statistics
Use numbers to describe your data:
- Mean, median, and mode
- Standard deviation
- Correlation between variables
5.3 Feature Engineering & Data Transformation
Create new features and change existing ones:
- Combine existing features
- Create categorical variables
- Scale numerical variables
- Introduction to Machine Learning
6.1 Types of Machine Learning: Supervised vs. Unsupervised
Understand different ways machines can learn:
- Supervised Learning: The computer learns from labeled examples
- Unsupervised Learning: The computer finds patterns on its own
6.2 Understanding Bias-Variance Tradeoff
Learn about the balance between simplicity and complexity in models:
- Bias: When a model is too simple
- Variance: When a model is too complex
- Finding the right balance
6.3 Data Splitting: Train-Test Split & Cross-Validation
Discover how to properly test your models:
- Train-Test Split: Divide data into training and testing sets
- Cross-Validation: Use multiple splits to get a better idea of model performance
- Supervised Learning Models
7.1 Regression Models: Linear Regression, Logistic Regression
Learn about models that predict numbers or categories:
- Linear Regression: Predict a number
- Logistic Regression: Predict a category (usually yes/no)
7.2 Classification Models: Decision Trees, Random Forest, SVM
Explore more ways to predict categories:
- Decision Trees: Make decisions based on features
- Random Forest: Combine many decision trees
- SVM (Support Vector Machine): Find the best line to separate categories
7.3 Model Evaluation Metrics
Learn how to measure how well your models are doing:
- Accuracy: How often the model is correct
- Precision: How often the model is right when it predicts positive
- Recall: How many positive cases the model finds
- F1-Score: A balance between precision and recall
- Unsupervised Learning Models
8.1 Clustering Techniques: K-Means, Hierarchical Clustering
Discover ways to group similar data points:
- K-Means: Group data into a set number of clusters
- Hierarchical Clustering: Create a tree-like structure of clusters
8.2 Dimensionality Reduction: PCA, t-SNE
Learn how to simplify your data while keeping important information:
- PCA (Principal Component Analysis): Find the most important features
- t-SNE: Visualize high-dimensional data in 2D or 3D
- Feature Selection & Model Optimization
9.1 Feature Scaling & Normalization
Prepare your data for better model performance:
- Scaling: Make all features have similar ranges
- Normalization: Change the distribution of your data
9.2 Hyperparameter Tuning using GridSearchCV & RandomizedSearchCV
Find the best settings for your models:
- GridSearchCV: Try all combinations of settings
- RandomizedSearchCV: Try random combinations of settings
- Deep Learning Basics with TensorFlow & Keras
10.1 Introduction to Neural Networks
Learn about a powerful type of machine learning:
- Neurons and layers
- Activation functions
- Backpropagation
10.2 Building a Simple Deep Learning Model
Create your first neural network:
- Define the structure
- Compile the model
- Train and evaluate
10.3 CNNs for Image Recognition
Explore neural networks that work well with images:
- Convolutional layers
- Pooling layers
- Image classification tasks
- Working with Real-World Datasets & Projects
11.1 Hands-on Project 1: Predicting House Prices
Apply what you’ve learned to a real problem:
- Load and clean housing data
- Create features
- Build and evaluate a regression model
11.2 Hands-on Project 2: Customer Segmentation using Clustering
Group customers based on their behavior:
- Prepare customer data
- Apply clustering algorithms
- Interpret the results
11.3 Hands-on Project 3: Sentiment Analysis using NLP
Analyze text data to understand opinions:
- Process text data
- Create features from text
- Build a sentiment classification model
- Deploying Machine Learning Models
12.1 Saving & Loading Models with Pickle & Joblib
Learn how to save your models for later use:
- Save models to files
- Load models from files
12.2 Model Deployment using Flask / FastAPI
Make your models available as web services:
- Create a simple web application
- Connect your model to the application
- Handle requests and responses
12.3 Deploying on Cloud (AWS, Google Cloud, Heroku)
Make your models available to everyone:
- Choose a cloud provider
- Set up your environment
- Deploy your application
- Advanced Topics
13.1 Time Series Forecasting
Learn how to work with data that changes over time:
- Understand time series data
- Use models specific to time series
- Make predictions about the future
13.2 Natural Language Processing (NLP)
Explore how to work with text data:
- Tokenization and stemming
- Part-of-speech tagging
- Named entity recognition
13.3 Reinforcement Learning Basics
Discover how to create systems that learn by interacting with an environment:
- Agents and environments
- Rewards and policies
- Q-learning
- Career Guidance & Certifications
14.1 Data Science Certifications
Learn about ways to prove your skills:
- Google Data Analytics Professional Certificate
- IBM Data Science Professional Certificate
- Coursera Data Science Specializations
14.2 Resume Building & Interview Preparation
Get ready to apply for Data Science jobs:
- Create a strong resume
- Prepare for technical interviews
- Practice explaining your projects
14.3 Freelancing & Job Opportunities in Data Science
Explore different ways to work in Data Science:
- Full-time positions
- Freelance projects
- Data Science competitions
- Conclusion & Next Steps
15.1 Best Practices for Data Science Projects
Learn how to do great work:
- Document your code
- Version control with Git
- Reproducible research
15.2 How to Stay Updated in the Data Science Field
Keep learning and growing:
- Follow Data Science blogs and news
- Attend conferences and meetups
- Contribute to open-source projects
This guide covers the main topics you need to learn Data Science with Python. Remember to practice regularly and work on your own projects to really understand these concepts.
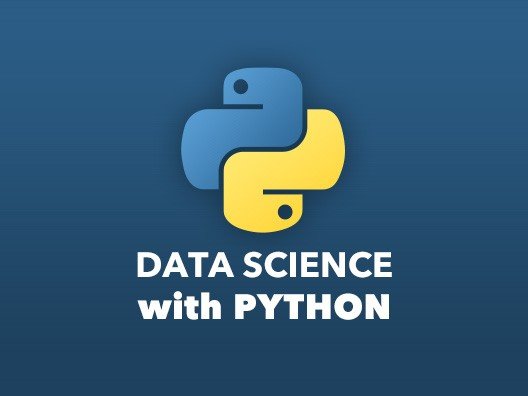